9. C Program to find Simple Interest and Compound Interest.
How does this program work?
- In this programme you will learn about how to calculate Simple Interest and Compound Interest.
- This programme requires the user input to enter the principle amount, time and rate of interest.
- This programme perform mathematical calculation and display the result using C language.
Here is the code
#include <stdio.h>
#include <math.h>
int main()
{
//Program to find the simple interest and compound interest
int p,t;
float r,si,amount,ci;
printf("Please enter principle,time and rate of interest");
scanf("%d%d%f",&p,&t,&r);
si=p*t*r/100;
//Simple Interest formula is p*t*r
printf("\nSimple interest = %.3f",si);
//Compound Interest formula is below
amount=p*pow((1 +r/100),t);
ci=amount-p;
printf("\nCompound interest = %.3f",ci);
return 0;
}
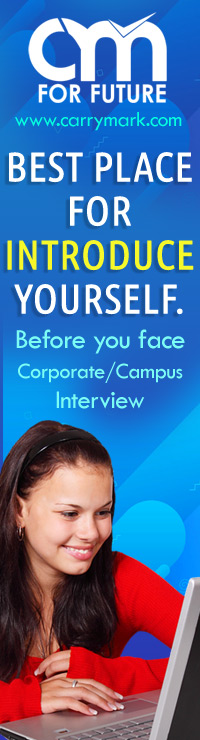