177. C Program to Store Student Details Using Structures.
How does this program work?
- This Program is used to store student details using Structures.
Here is the code
#include <stdio.h>
struct student {
char name[30];
int roll;
float perc;
};
int main()
{
//Program to read the student number, name, average score and
//Print these details with appropriate grades by using pointers to structures
struct student std; //Structure variable
struct student *ptr; //Pointer to student structure
ptr= & std; //Assigning value of structure variable
printf("Enter details of student: ");
printf("\n Enter your Name :");
gets(ptr->name);
printf("\nEnter Roll No:");
scanf("%d",&ptr->roll);
printf("Enter Percentage ?:\n");
scanf("%f",&ptr->perc);
printf("\nEntered details: ");
printf("\nName:%s \nRollNo: %d \nPercentage: %.02f\n", ptr->name, ptr->roll, ptr->perc);
return 0;
}
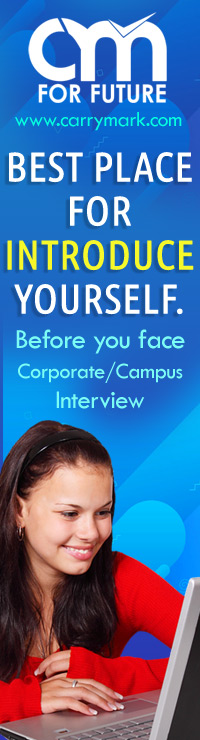