27. C Program To Check Whether The Given Year is Leap Year or Not.
How does this program work?
- In This program your going to learn about how to find the given year is leap year or not.
- By using If else condition here we check the condition for leap year.
- If the given year is exactly divisble by 4 and 400.
- It is denoted as leap year else it not a leap year.
Here is the code
#include <stdio.h>
int main(void)
{
int year;
printf("Enter a year to check it is a leap year or not\n");
scanf("%d", &year);
// If Exactly divisible by 400 e.g. 1600, 2000
if (year%400 == 0)
printf("%d is a leap year.\n", year);
//If Exactly divisible by 100 and not by 400 e.g. 1900, 2100
else if (year%100 == 0)
printf("%d is not a leap year.\n", year);
//If Exactly divisible by 4 and neither by 100 nor 400 e.g. 2020
else if (year%4 == 0)
printf("%d is a leap year.\n", year);
else
// Not divisible by 4 or 100 or 400 e.g. 2017, 2018, 2019
printf("%d is not a leap year.\n", year);
return 0;
}
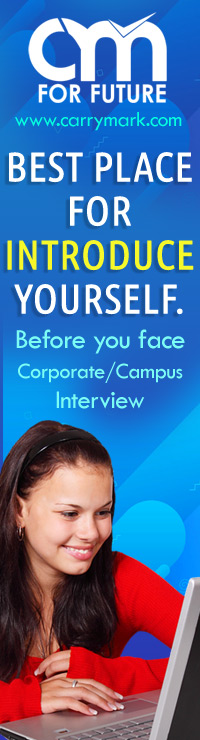