28. C Program To Find Roots for the Given Quadratic Equation.
How does this program work?
- In this program you are going to learn about how to find roots for the given quadratic equation.
-
The term b2-4ac is known as the discriminant of a quadratic equation. The discriminant tells the nature of the roots.
- If discriminant is greater than 0, the roots are real and different.
- If discriminant is equal to 0, the roots are real and equal.
- If discriminant is less than 0, the roots are complex and different.
- In this program, library function sqrt() is used to find the square root of a number. To learn more, visit: sqrt() function.
Here is the code
#include <stdio.h>
#include <math.h>
int main(void)
{
float a,b,c;
float d,root1,root2;
printf("Enter a, b and c of quadratic equation: \n");
scanf("%f%f%f",&a,&b,&c);
d = b * b - 4 * a * c; // Discriminant of a quadratic equation
if(d < 0)
{
printf("Roots are complex number.\n");
printf("Roots of quadratic equation are: \n");
printf("%.3f%+.3fi",-b/(2*a),sqrt(-d)/(2*a));
printf(", %.3f%+.3fi",-b/(2*a),-sqrt(-d)/(2*a));
return 0;
}
else if(d==0)
{
printf("Both roots are equal.\n");
root1 = -b /(2* a);
printf("Root of quadratic equation is: %.3f \n",root1);
return 0;
}
else
{
printf("Roots are real numbers.\n");
root1 = ( -b + sqrt(d)) / (2* a);
root2 = ( -b - sqrt(d)) / (2* a);
printf("Roots of quadratic equation are: %.3f , %.3f\n",root1,root2);
}
return 0;
}
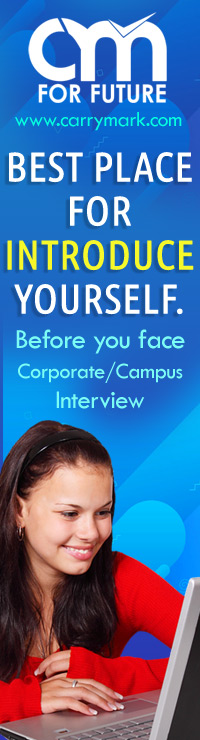