193. C Program to Construct Book using Structures.
How does this program work?
- This Program is used to construct book using structures.
Here is the code
#include <string.h>
struct books
{
char title[50];
char author[50];
char subject[50];
int book_id;
};
int main(void)
{
struct books Book1;
struct books Book2;
strcpy(Book1.title,"Mathematics");
strcpy(Book1.author,"sowmya");
strcpy(Book1.subject,"Maths");
Book1.book_id="252";
strcpy(Book2.title,"Softwere");
strcpy(Book2.author,"Gowthami");
strcpy(Book2.subject,"programming");
Book2.book_id="435";
printf("Book1 title:%s\n",Book1.title);
printf("Book1 author:%s\n",Book1.author);
printf("Book1 subject:%s\n",Book1.subject);
printf("Book1 book_id:%d\n",Book1.book_id);
printf("Book2 title:%s\n",Book2.title);
printf("Book2 author:%s\n",Book2.author);
printf("Book2 subject:%s\n",Book2.subject);
printf("Book2 book_id:%d\n",Book2.book_id);
return 0;
}
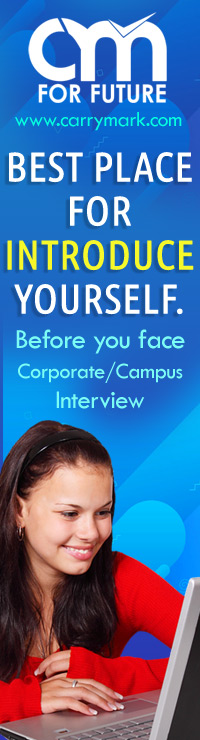