194. C Program to Display The Student Details using Structures.
How does this program work?
- This Program is used to display the student details using structures.
Here is the code
//Initialize structure
struct Student
{
int Roll;
char Name[25];
int Marks[3];
int Total;
float Avg;
};
int main(void)
{
int i;
struct Student S;
printf("Enter Student Roll : \n");
scanf("%d",&S.Roll);
printf( "\n\nEnter Student Name : \n");
scanf( "%s",&S.Name);
S.Total = 0;
//To compute 3subjects total Marks
for(i=0;i<3;i++)
{
printf("Enter Marks %d :\n ",i+1);
scanf("%d",&S.Marks[i]);
S.Total = S.Total + S.Marks[i];
}
//To find Average of 3marks
S.Avg = S.Total / 3;
printf("\nRoll : %d",S.Roll);
printf("\nName : %s",S.Name);
printf("\nTotal : %d",S.Total);
printf("\nAverage : %f",S.Avg);
return 0;
}
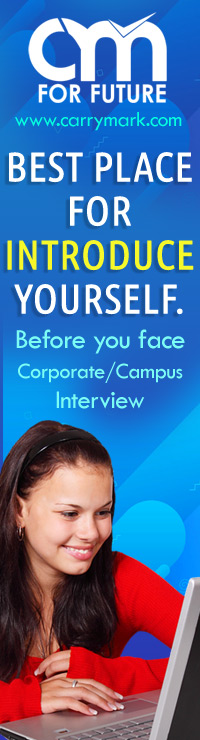