139. C Program to print the Outer Square of the given matrix.
How does this program work?
- In this programme you will learn about how to find the outer square of the given matrix.
- Matrix elements are stored in array variables.
Here is the code
#include <stdio.h>
void printBoundary(int a[][4], int m, int n)
{
for(int i = 0; i < m; i++)
{
for (int j = 0; j < n; j++)
{
if(i == 0)
printf("%d",a[i][j] );
else
if (i == m - 1)
printf("%d",a[i][j]);
else
if(j == 0)
printf("%d",a[i][j]);
else
if(j == n - 1)
printf("%d",a[i][j]);
else
printf(" ");
}
printf("\n");
}
}
// Driver program to test above function
int main(void)
{
//Intialise matrix elements and rows and columns
int a[4][4] = { 1, 2, 3, 4 ,2,3,4,1,3,4,2,1,4,3,2,1} ;
printBoundary(a, 4, 4);
return 0;
}
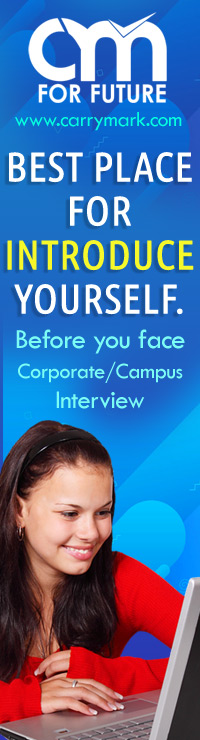