140. C Program to Transverse the given matrix Helically.
How does this program work?
- In this programme you will learn about to print the given matrix helically using C program.
- Matrix elements are stored in array variables.
Here is the code
#include <stdio.h>
void spiralPrint(int m, int n, int a[][n])
{
//153.Write a program to traverse the matrix helically
int i, k = 0, l = 0;
m--, n--;
while(k <= m && l <= n)
{
// Print the row left to right
for(i = l; i <= n; ++i)
{
printf("%d ", a[k][i]);
}
// Print the column top to bottom
k++;
for(i = k; i <= m; ++i)
{
printf("%d ", a[i][n]);
}
// Print the row right to left
n--;
if(m >= k)
{
for(i = n; i >= l; --i)
{
printf("%d ", a[m][i]);
}
m--;
}
// Print the column bottom to top
for(i = m; i >= k; --i)
{
printf("%d ", a[i][l]);
}
l++;
}
printf("\n");
}
int main(void)
{
int arr[3][3] = {1, 2, 3, 4, 5, 6, 7, 8,9};
spiralPrint(3, 3, arr);
return 0;
}
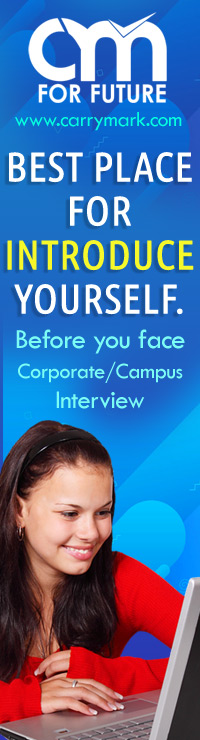