Python Theory
Arrays:
Or
Arrays elements are mutable which means they are changeable i.e we can add elements and remove elements easily.
Is a python list the same as an array?
How to create arrays in python?
The array(data type,value list) takes two parameters.
Syntax |
---|
a =arr.array(data type,value List) or a =array(data type,value List) |
Example |
---|
import array a =array.array('i',[1,2,3,45,67,9]) print(a) import array as arr array =arr.array('i',[1,2,3,4,5,6]) print(a) from array import * a =array('i',[1,2,3,4,5,6]) print(a) |
Code | C code | Python Data Type | Byte |
---|---|---|---|
i | Signed int | int | 2 |
I | Unsigned int | Int | 2 |
u | unicode | unicode | 2 |
H | Unsigned short | Unsigned short | 2 |
h | Signed short | Signed short | 2 |
l | Signed long | Signed long | 4 |
L | Unsigned long | Unsigned long | 4 |
f | Float | Float | 4 |
d | Double | Float | 4 |
Accessing array elements:
Syntax |
---|
Array_name[index value] |
Example |
---|
import array as arr a =arr.array('i',[4,3,5,7]) print("First element:", a[0]) print("Last element:", a[-1]) |
Output |
---|
|
Slicing Python Arrays:
An array can be sliced using the : symbol. This returns a range of elements that we have specified by the index numbers.
Example |
---|
import array as arr a =arr.array('i',[4,3,5,7]) print(a[1:3]) print(a[:-2]) print(a[:]) |
Output |
---|
|
Finding the length of the array
Syntax |
---|
len(arry_name) |
Example |
---|
import array as arr a =arr.array('i',[1.1,2.1,3.1]) len(a) |
Changing and Adding Elements:
Example |
---|
import array as arr a =arr.array('i',[1,2,3,4,5]) #changing first element a [0]=0 print(a) #changing 2nd and 4th element a [1:5]=arr.array('i',[4,6,8]) print(a) |
Output |
---|
|
Adding elements to an array:
Method | Description |
---|---|
append() | Used when you want to add a single element at the end of an array. |
extend() | Used when you want to add more than one element at the end of an array. |
Insert() | Used when you want to add an element at a specific position in an array |
Example |
---|
import array as arr a =arr.array('i',[1,2,3]) a.append(4) print(a) b =arr.array('d',[1.1,2.3]) b.extend([1.5,3]) print(b) c =arr.array('i',[9,3,4]) c.insert(2,34) print(c) |
Output |
---|
|
Removing elements of an array:
Method | Description |
---|---|
pop() | Used when you want to remove an element and return it. |
remove() | Used when you want to remove an element with a specific value without returning it . |
Example |
---|
import array as arr a =arr.array('d',[1,2,3,4,5]) a.pop( ) print(a) a.pop(3) print(a) a.remove(2) print(a) |
Output |
---|
|
Array concatenation:
Concatenation two arrays Using “+” symbol
Example |
---|
# Concatenation of two arrays import array as arr a =arr.array('i',[1,3,5]) b =arr.array('i',[2,4,6]) c=a+b print(c) |
Output |
---|
|
Looping through array:
We can loop through an array easily using the for and while loops
Method | Description |
---|---|
for() | Iterates over the items of an array specified number of times. |
while() | Iterates over the elements until a certain condition is met. |
Example |
---|
import array as arr a =arr.array('d',[1,2,3,4,5]) print("All Values:") for x in a: print(x) |
Output |
---|
|
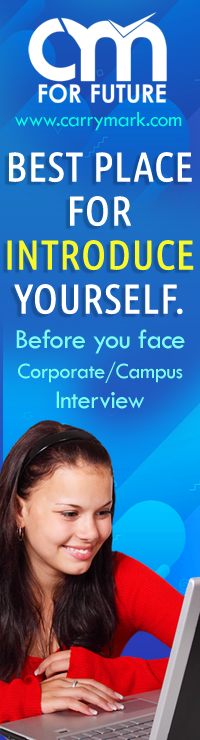