Python Theory
Control Statements (Looping Statements):
The following are the loop structures available in python.
While Statement:
Syntax |
---|
while expression: statement(s) |
Example |
---|
n =10 # initialize sum sum =0 i =1 while i <=n: sum =sum+i i =i+1 print(" The sum is", sum) |
Using else statement with while loops
Example |
---|
counter =0 while counter < 3: print("Inside loop ") counter = counter + 1 else: print("Inside else ") |
Output |
---|
|
For Loop Statement:
Syntax |
---|
for val in sequence: Body of for loop |
Example |
---|
# List of numbers numbers = [ 2,4,6,8,10 ] # variable to store the sum sum= 0 # iterate over the list for val in numbers: sum= sum+val print("The sum is ", sum) |
Using else statement with file loops:
Example |
---|
digits = [ 0,2,5 ] for i in digits: print( i ) else: print("No items left.") |
Output |
---|
|
Range() function:
The range() function is used for a sequence of numbers, starting from 0 by default 1 and increment by 1.
Syntax |
---|
range(start, stop, step) |
Example |
---|
# Defining Range print(range(5)) print(list (range(5))) print(list (range(1,9))) print(list (range(2,10,3))) |
Output |
---|
|
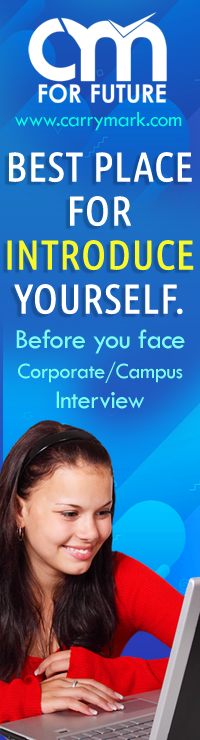