Python Theory
Operators:
An operator is a symbol that represents an operation that may be performed on one or more operands.
An operand is a value that a given operator is applied to.
Types of operators in python:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Membership Operators
- Bitwise Operators
Arithmetic Operators:
Arithmetic Operators are used to perform arithmetic operations between two operands.
Operator | Description | Example |
---|---|---|
+ | Add two operators | A+B (2+3=5) |
- | Subtract second operand from the first | A-B(9-3=6) |
* | Multiplies both operators | A*B(2*4=8) |
/ | Divides numerator by denominator | A/B(10/2=5) |
// Floor Division | Divide and return the integer value of the quotient. | A//B(12//10=1) |
% Modulus | Modulus operators and remainder of after an integer division. | A%B(10%2=0) |
** Exponentiation | left operand raised to the power of right. | A**B(10**2=200) |
Assignment Operators:
Assignment Operators are used to assign values to variables.
Operator | Description | Example |
---|---|---|
= | Assign a value. | A=5 |
+= | Add and assign the result to the variable. | a+=1(a=a+1) |
-= | Subtract and assign the result to the variable. | a-=1(a=a-1) |
*= | Multiple and assign the result to the variable. | a*=5(a=a*5) |
/= | Division and assign the result to the variable. | a/=5(a=a/5) |
//= | Floor division and assign the result to the variable. | a//=6(a=a//6) |
%= | Modulus and assign the result to the variable. | a%=5(a=a%5) |
**= | Exponentiation and assign the result to the variable. | a**=5(a=a**5) |
&= | Bitwise AND and assign the result to the variable. | a&=5(a=a&5) |
|= | Bitwise OR and assign the result to the variable. | a|=5(a=a|5) |
^= | Bitwise XOR and assign the result to the variable. | a^=5(a=a^5) |
>>= | Bitwise right shift and assign the result to the variable. | a>>=5(a=a>>5) |
<<= | Bitwise left shift and assign the result to the variable. | a<<=5(a=<<5) |
Relational Operators:
1. Relations Operators are also called as comparison operators.
2. It is used to compare values.
3. It either returns TRUE or FALSE according to the condition.
Operator | Description | Example |
---|---|---|
> | Greater than | A>B |
< | Less than | A< B |
== | Equal to | A==B |
!= | Not Equal to | A!=B |
>= | Greater than or equal to | A>=B |
<= | Less than or equal to | A<=B |
Logical Operators:
Logical Operator are the and,or, not operator.
Operator | Description | Example |
---|---|---|
AND | True if both the operands are true | 10< 5 and 10< 20 (FALSE) |
OR | True if either of the operands is true | 105 or 10< 20(TRUE) |
NOT | True if operand is false | not(10< 20) FALSE |
Membership Operators:
Python offers some special operators like identity operators and the membership operators.
1. Identity Operator:
Is and Is not are the identity operator.
Operator | Description | Condition | Example |
---|---|---|---|
is | True if the operands are identical | A is true | x=2y=2Z=3X is Y (TRUE) |
Is not | True if the operands are not identical | A is not true | X is not Z |
2. Membership Operator:
In and not In are the membership operators.
Operator | Description | Condition | Example |
---|---|---|---|
in | True if value/variable is found in the sequence. | 5 in a | x=’skill pundit’ y = {1:'a',2:'b'}S in x (TRUE) |
In not | True if value/variable is not found in the sequence | 5 in not a | S IN NOT Y(FALSE) |
Bitwise Operator:
1. Bitwise operator act on operands as if they are string of binary digits.
2. It operates bit by bit.
Operator | Description | Example |
---|---|---|
& | Bitwise AND | A&B |
| | Bitwise OR | A|B |
~ | Bitwise NOT | A~B |
^ | Bitwise XOR | A^B |
>> | Bitwise right shift | A>>B |
<< | Bitwise left shift | A<< B |
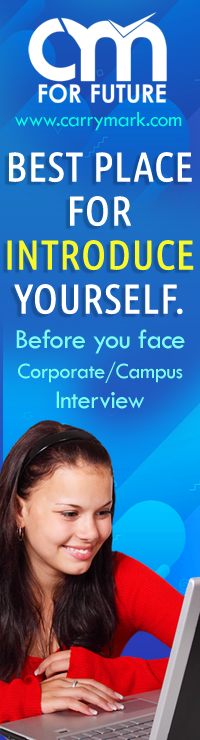