Python Theory
Conditional Statements (Decision Making):
if statement:
Syntax |
---|
if test expression: statement(s) |
Example |
---|
a=int(input("Enter the number:")) if (a<=10 ): print(" Condition is True") |
Output |
---|
|
If else statement :
The if else statement is called alternative execution, in which there are two possibilities and the condition determines which one gets executed.
Syntax |
---|
if test expression: statement(s) else: statement(s) |
Example |
---|
a=int(input("Enter the number:")) if (a<=10 ): print(" Condition is true") else: print(" Condition is Flase") |
elif statements:
Syntax |
---|
if expression: Body of if elif expression: Body of elif else: Body of else |
Example |
---|
a=int(input("Enter the number:")) b=int(input("Enter the number:")) c=int(input("Enter the number:")) if (a>b ) and (a>c ): print(" a is greater") elif (b>a ) and (b>c ): print(" b is greater") else: print(" c is greater") |
Nested if statement:
Syntax |
---|
if expression1: statement(s) if expression2: statement(s) elif expression3: statement(s) else: statement(s) |
Example |
---|
n=int(input("Enter the number:")) if (n<=15 ): if (n==10 ): print(" Play Cricket") else: print(" Play kabadi") else print(" Don't play game") |
Output |
---|
|
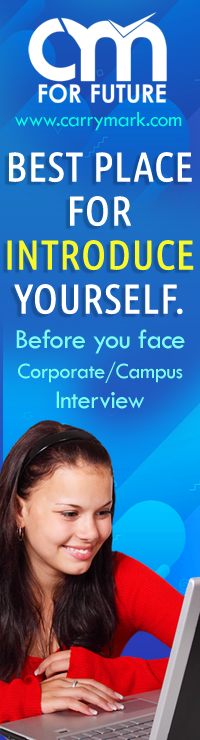