Python Theory
List:
List is a collection of values or items of different types .
Python lists are written with square brackets and each item in the list is seperated with comma(,).
A list can be defined as below:
L1=["python","program","indexing"]
L2=[1,2,3,5,8]
Example |
---|
#List Define L1=["python","program","indexing"] L2=[1,2,3,5,8] print(type(L1)) print(type(L2)) |
Output |
---|
|
Characteristics of lists:
- Lists are mutable type.
- Lists elements can be changable.
- Lists are ordered.
- Index in python starts from 0 not 1.
List-Indexing And Slicing:
Example |
---|
#List Indexing And Slicing list=["skillpundit","python","Rossum",435,234,333] print(list[1]) print(list[2:5]) print(list[0:4]) print(list[:]) #Negative Indexing list1=[1,2,3,4,5,6] print(list1[-1]) print(list1[-3:]) print(list1[:-3]) |
Output |
---|
|
List Methods:
1. Append()
Add an element to the end of the list.
2. Extend()
Add all elements of one list to the other list.
3. Insert()
Insert an item at the defined index.
Example |
---|
#List Append() And Extend() list=[1,2,3,4,5] list.append(6) print(list) list.extend([7,8,9]) print(list) #List Insert() list.insert(1,3) print(list) |
Output |
---|
|
4. Remove()
Removes an item from the list .
5. Pop()
Removes and returns an element at the given index.
Example |
---|
#Delete and Remove List list=['p','r','o','g','r','a','m','m','i','n','g'] del list[2] print(list) #Delete multiple items del list[1:3] print(list) list.remove('p') print(list) print(list.pop(1)) print(list) print(list.pop()) print(list) |
Output |
---|
|
6. Sort()
Sort items in a list in ascending order.
7. Index()
Returns the index of the first matched item.
8. Count()
Returns the count of the number of items passed as an argument.
9. Reverse()
Reverse the order of items in the list.
Example |
---|
#List Methods list=[1,3,5,7,9,11,13,3] print(list.index(9)) print(list.count(3)) list.sort() print(list) list.reverse() print(list) |
Output |
---|
|
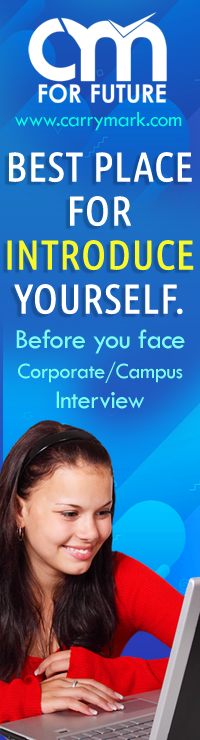