Python Theory
Strings:
Example |
---|
# Defining strings in Python string = 'skillpundit' print(string) string1 = "skillpundit" print(string1) string2 = '''skillpundit''' print(string2) # triple quotes string can extend multiple lines string3 = """HI, welcome to skillpundit""" print(string3) |
Output |
---|
|
String Indexing:
String Slicing:
H | E | L | L | O |
---|---|---|---|---|
0 | 1 | 2 | 3 | 4 |
-5 | -4 | -3 | -2 | -1 |
Syntax |
---|
string _variablename [start:end] |
Example |
---|
#Accessing string characters in Python str = 'skillpundit' print('str = ', str) #frist character print('str [0] =', str[0]) #last character print('str [-1] =', str[-1]) #slicing 2nd to 5th character print('str [1:5] =', str[1:5]) #slicing 5th to 2nd last character print('str [4:-2] =', str[5:-2]) print('str [0:15:2 ] =', str[0:11:2]) |
Output |
---|
|
Strings Concatenation:
Example |
---|
#Python String Operations str1 = ' welcome to ' str2 = 'skillpundit' # using + print('str1 + str2 = ', str1 +"" +str2) # using * print('str2 * 2 = ', str2 * 2) |
Output |
---|
|
Strings Functions and Methods:
len() | min() | max() | isalnum() | isalpha() |
isdigit() | islower() | isupper() | isspace() | isidentifier() |
endswitch() | startswitch() | find() | count() | capitalize() |
title() | lower() | upper() | swapcase() | replace() |
center() | ljust() | rjust() | center() | isstrip() |
rstrip() | strip() |
1. Converting string functions:
capitalize() | Only the first character capitalized. |
lower() | All characters converted to lowercase. |
upper() | All characters converted to uppercase. |
title() | First character capitalized in each word. |
swapcase() | Lower case letters are converted to uppercase and uppercase letters are converted to lowercase. |
replace(old,new) | Replaces old string with new string. |
Example |
---|
#string functions str = "welcome to skillpundit" print("Capitalize:", str.capitalize()) print("Lowercase:", str.lower()) print("Uppercase:", str.upper()) print("Titlecase", str.title()) print("Swapcase:", str.swapcase()) print("Replacecase:", str.replace("skillpundit","python")) |
Output |
---|
|
2. Formatting string functions:
center(width) | Returns a string centered in a field of given width. |
ljust(width) | Returns a string left justified in a field of given width. |
rjust(width) | Returns a string right justified in a field of given width. |
format(items) | Format a string. |
Example |
---|
#Formatting string Functions: a = input("Enter String:") print("Center Alignment:", a.center(20)) print("Left Alignment:", a.ljust(20)) print("Right Alignment:", a.rjust(20)) |
Output |
---|
|
3. Removing whitespace Characters:
lstrip() | Returns a string with leading and trailing whitespace characters removed. |
rstip | Returns a string with whitespace characters removed. |
strip() | Returns a string with trailing whitespace characters removed. |
4. String Modules :
Mostly used string modules:
- string.upper()
- string.lower()
- string.split()
- string.join()
- string.replace()
- string.count()
- string.find()
Example |
---|
a = 'skillpundit' b = a.capitalize() print(b) b = a.count('i') print(b) b = a.find('u') print(b) b = a.index('d') print(b) b = a.isalnum( ) print(b) b = a.lower( ) print(b) b = a.upper( ) print(b) b = a.strip( ) print(b) |
Output |
---|
|
Strings are Immutable:
Example |
---|
a = 'python program' print(a[0]) print(a[8]) a [0]= "b" |
Output |
---|
|
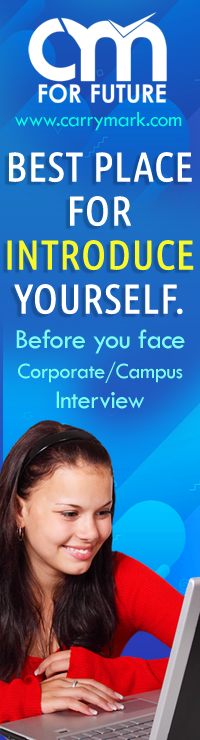