Python Theory
Dictionary:
Declaration:
dictionary = {'key': 'value1', 'key2': 'value2', 'key3': 'value3'}Example |
---|
# Empty Dictionary dict = {} # Dictionary with integer keys dict = {1: 'Apple', 2: 'Graphs'} # Dictionary with mixed keys dict = {'name': 'skillpundit', 1: [4, 3, 5]} |
Output |
---|
|
Dictionary vs List
Dictionary | List |
---|---|
unordered | Ordered |
Accesses via keys | Accessed via index |
Collection of key value pairs. | Collection of elements. |
Preferred when we have unique key values. | Preferred for ordered data |
No Duplicate Members | Allows Duplicate Members |
Changing and Adding Dictionary Elements:
Add new items or change the value of existing items using an assignment operator.
Example |
---|
# Changing And Adding Dictionary Elements a = {1: 'Apple', 2: 'Graphs', 3: 'Banana'} # update value a[3] = 'Mango' print(a) # add item a[4] = 'Orange' print(a) |
Output |
---|
|
Operations in Python Dictionary:
Method | Description |
---|---|
clear() | Remove all items from the dictionary. |
copy() | Returns a copy of the dictionary. |
values() | Return all the values inside the dictionary. |
update() | Updates the values of the dictionary with specified key value pairs. |
get() | Returns the values of the key. |
items() | Return a new object of the dictionary's items in (key, value) format. |
keys() | Returns all the keys inside dictionary. |
pop() | Pop the value with the specified key. |
popitem() | The popitem() method removes the last inserted item in dictionary. |
Example |
---|
#Removing Elements From a Dictionary #Create Dictionary a = {1:1,2:4,3:9,4:16,5:25} #Remove a Particular Item #Output: 16 print(a.pop(4)) print(a) #Remove an arbitrary Item #Output: (5,25) print(a.popitem()) print(a) #Remove all items a.clear() #Output: {} print(a) #Delete the Dictionary del a #Throws Error print(a) |
Output |
---|
|
Example |
---|
#Dictionary Methods #Create a Dictionary a = {1:1 ,2:4 ,3:9 ,4:16 ,5:25} #List of all the Values in the dictionary print(a.values()) #items() print(a.items()) #get() print(a.get(3)) #keys print(a.keys()) #setdefault print(a.setdefault(5)) |
Output |
---|
|
Loops through Dictionary:
Loops through dictionary by using a for loop
Example |
---|
#Loops Through Dictionary #Create a Dictionary a = {1:1, 2:4, 3:9} for X in a: print(a[X]) for X,Y in a.items(): print(X,Y) |
Output |
---|
|
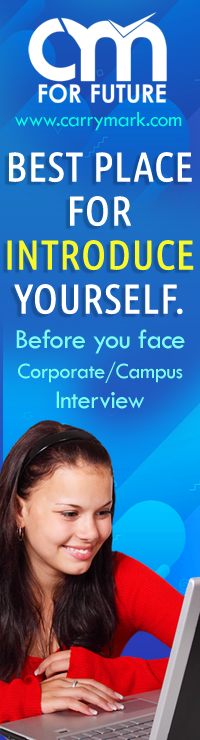