Python Theory
Tuple:
A tuple can be defined as below:
Example |
---|
#Different types Of tuples tuple = (1,2,3,4,5) print(tuple) tuple1 = ('Hello',3.5,5) print(tuple1) tuple1 = ('Apple',[2,4,8],[4,7,9]) print(tuple2) |
Output |
---|
|
Tuple indexing and Slicing:
The indexing and slicing in the tuple are similar to lists.
p | u | n | d | i | t |
---|---|---|---|---|---|
0 | 1 | 2 | 3 | 4 | 5 |
-6 | -5 | -4 | 3 | -2 | -1 |
Tuple[0]=p | Tuple[0:]=('p', 'u', 'n', 'd', 'i', 't') |
Tuple[1]=u | Tuple[:]=('p', 'u', 'n', 'd', 'i', 't') |
Tuple[2]=n | Tuple[:4]=('p', 'u', 'n', 'd') |
Tuple[3]=d | Tuple[2:4]=('n', 'd') |
Tuple[4]=i | |
Tuple[5]=t |
Two methods are available in Tuple:
Method | Description |
---|---|
count() | Returns the count of the number of items passed as an argument. |
index() | Returns the index of the first matched item. |
Example |
---|
tuple = ('A','P','P','L','L') print(tuple.count('P')) print(tuple.index('1')) |
Output |
---|
|
Advantages of list vs tuple
List | Tuple |
---|---|
List is a collection of arrays . | It is a collection of objects much like a list. |
The list is mutable. | The tuple is immutable. |
It represent as [] | It represent as () |
The lists are less memory efficient than a tuple. | The tuples are more efficient because of its immutability. |
List elements are changeable and ordered. | Tuples elements cannot be changed. |
The list has the variable length. | Tuple has a fixed length. |
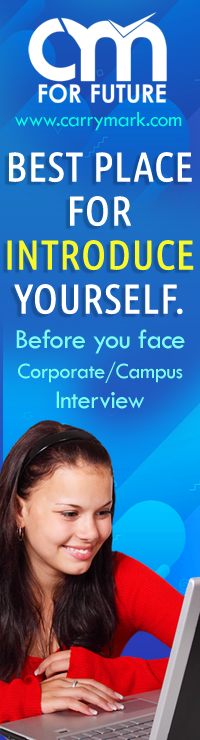