Python Theory
Sets:
Creating Python Sets:
A set is created by inside curly braces {}, separated by comma, or by using the built-in set() function.
Example |
---|
# Make set from a list set3 = set([1,2,3,2]) print(set3) # Set and Dictionary while creating empty set # Initialize a with {} a = {} print(type(a)) a = set() print(type(a)) |
Output |
---|
|
Example |
---|
# Different types of sets in Python # set of integers set3 = {1,2,3} print(set) # Set of mixed datatypes set1 = {1.0, "Hello", (1,2,3)} print(set1) # set cannot have duplicates set2 = {1,2,3,4,3,2} print(set2) |
Output |
---|
|
Modify sets in python:
We can add a single element using add() method, and multiple elements using the update() method.
Method | Description |
---|---|
add() | Add a single element using add() method. |
update() | Multiple elements using the update() method. |
discard() | |
remove() | |
pop() | Remove the last element in the set. |
clear() | The clear() method empties the set |
Example |
---|
#Adding Elements to the set set = {1,2} set.add(3) print(set) #Update Elements to the set set.update([4,5,6]) print(set) |
Output |
---|
|
Example |
---|
#discard() and remove() #initialize my_set my_set={1,3,4,5,6} print(my_set) #discard() an element my_set.discard(5) print(my_set) #remove an element my_set.remove(6) print(my_set) |
Output |
---|
|
Example |
---|
#initialize my_set set = set("skillpundit") print(set) #pop an element # output : random element print(set.pop()) #clear my_set set.clear() print(set) |
Output |
---|
|
Loop sets:
Iterating Through a set :
Example |
---|
set = {"apple", "banana", "cherry"} for X in set: print(X) |
Output |
---|
|
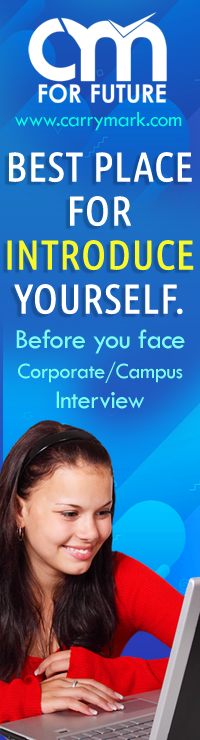