Python Theory
Variables in Python:
A variable is a name which is associated with a value that can be changed. It is a name of memory location.
Rules for python variables:
- Variable names must start with a letter or the underscore character.
- Variable names cannot start with a number.
- A variable name can only contain alphanumeric characters and underscores (A-z, 0-9, and _ ).
- Variable names are case-sensitive (age, Age and AGE are three different variables).
Variable Declaration :
x=5
y= “john”
print(x)
print(y)
Examples: |
---|
x=10
y=20 print(x+y) print(x*y) |
Data types:
Data types specifies the type of values that can be stored in the variable.
Example | Datatype |
---|---|
X = 20 | int |
X = 20.5 | float |
X = 20j | complex |
X = “Hello world” | string |
X = ["apple", "banana","cherry"] | list |
X = ("apple", "banana","cherry") | tuple |
X = {"name" : "John", "age" :36} | dictionary |
X = {"apple", "banana","cherry"} | set |
Numbers:Numbers or Numerics mainly are numeric values
Examples: Integers: x=10 type(x) output: <class 'int'> float: x=10.5 type(x) output: <class 'float'> Complex: x=10j type(x) <class 'complex'> |
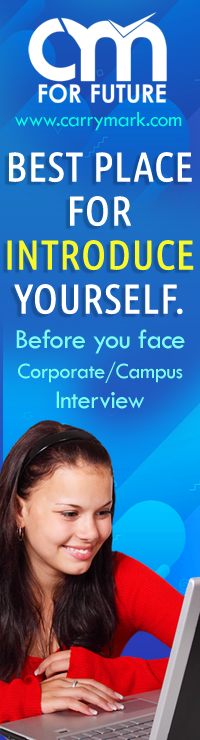