15. Python Program to find the Distance between given two points.
How does this program work?
- In this program you will learn about how to find the distance between two points by using given X, Y co-odinates.
- First we need to store user entered values in x1, y1 and x2, y2.
- After that by using the given formula we can get the distance between two points by using Python.
Here is the code
import math
def caldist(x1,y1,x2,y2):
#Distance between two points (D = sqrt((x2-x1)^2 + (y2-y1)^2))
distance = math.sqrt((x2 - x1) ** 2 + (y2 - y1) ** 2)
return distance
x1 = int(input("Enter the first coordinate of first point: "))
y1 = int(input("Enter the second coordinate of first point: "))
x2 = int(input("Enter the first coordinate of second point: " ))
y2 = int(input("Enter the second coordinate of second point: " ))
print("Distance between two points is: ",caldist(x1, y1, x2, y2))
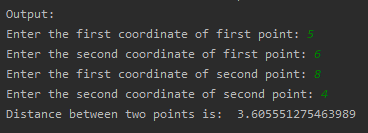
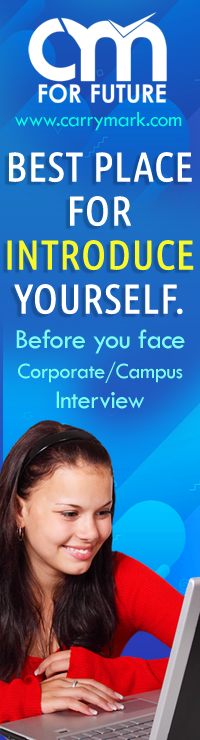