9. Python Program to find Simple Interest and Compound Interest.
How does this program work?
- In this program you will learn about how to find Simple Interest and Compound Interest using Python.
- This program requires the user input to enter the principle amount, time peroid and rate of interest.
- And then by using the given logic you will get the output.
Here is the code
#To Calculate Compound Interest
def compound_interest(principle, rate, time):
result = principle * (pow((1 + rate / 100),time))
return result
#To Calculate Simple Interest
def simple_interest(principle, rate, time):
result = (principle * rate * time)/100
return result
p = float(input("Enter the principal amount: "))
r = float(input("Enter the interest rate:" ))
t = float(input("Enter the time in years: "))
amount = compound_interest(p, r, t)
interest = amount - p
simple_int = simple_interest (p, r, t)
print("Compound amount is %.2f " % amount)
print("Compound interest is %.2f " % interest)
print("Simple interest is %.2f " % simple_int)
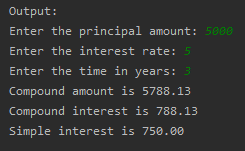
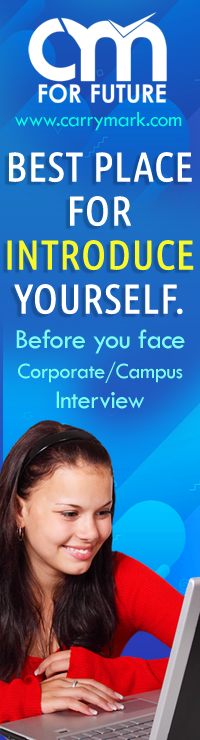