141. Python Program to check given Matrix is Magic Square or Not.
How does this program work?
- In this program we are going to learn about how to check whether the given matrix is Magic Squre or not using Python.
- In this program we calculated row sum, column sum and diagonal sum of distinct elements of the given matrix.
- If these sums are equal then print given matrix is magic square otherwise it is not a magic square matrix.
Here is the code
def MagicSquare( a,n) :
s = 0
for i in range(0, n) :
s = s + a[i][i]
s2 = 0
for i in range(0, n) :
s2 = s2 + a[i][n-i-1]
if(s!=s2) :
return False
for i in range(0, n) :
rowSum = 0;
for j in range(0, n) :
rowSum += a[i][j]
if (rowSum != s) :
return False
for i in range(0, n):
colSum = 0
for j in range(0, n) :
colSum += a[j][i]
if (s != colSum) :
return False
return True
a=[]
n=int(input("Enter the size of the matrix: "))
print("Enter the elements: ")
for i in range(n):
row=[]
for j in range(n):
row.append(int(input()))
a.append(row)
print(a)
print("Display an array In Matrix Form: ")
for i in range(n):
for j in range(n):
print(a[i][j], end=" ")
print()
if (MagicSquare(a,n)) :
print( "Magic Square")
else :
print( "Not a magic Square")
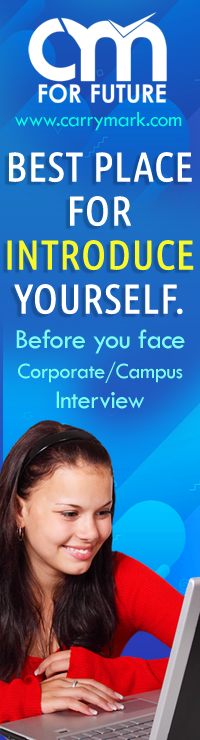