142. Python Program to Find the Saddle Point of the given Matrix.
How does this program work?
- In this program we are going to learn about how to find the Saddle point of the matrix using Python.
- Saddle point means minimum element in row is equal to the maximum element in column of a matrix.
- Find the minimum element in its row and maximum element in its column.
- Check if row minimum element and column maximum element. If it is equal then print saddle point, otherwise no saddle point present in given matrix
Here is the code
def findSaddlePoint(mat, n):
for i in range(0,n):
min_row = mat[i][0]
col_ind = 0
for j in range(1,n):
if (min_row > mat[i][j]):
min_row = mat[i][j]
col_ind = j
for k in range(0,n):
if (min_row < mat[k][col_ind]):
break
if (k == n):
print("Value of Saddle Point: ", min_row)
return True
return False
mat= [[1, 2, 3],
[4, 5, 6],
[5,4, 6]]
n = 3
print("Given matrix is : ")
print(mat)
if (findSaddlePoint(mat, n) == False):
print("No Saddle Point ")
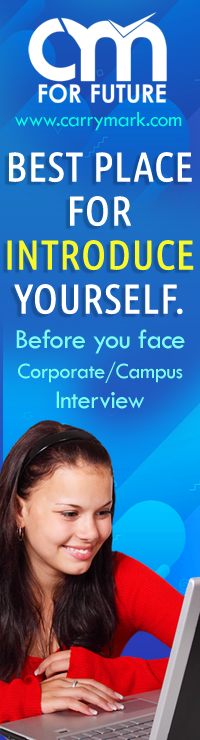