145. Python Program to find the Factorial of a given number using Recursion.
How does this program work?
- This program is used to find the factorial of a given number using recursion in Python.
- Factorial number is defined by the product of all the digits of given number.
- Factorial of 0 is always 1.
- Recursion method is used to find the factorial of a given number.
Here is the code
def fact(n):
if n == 1:
return n
else:
return n*fact(n-1)
num = int(input("Enter a number: "))
if num < 0:
print("Sorry, factorial does not exist for negative numbers")
elif num == 0:
print("The factorial of 0 is 1")
else:
print("The factorial of ",num," is ",fact(num))
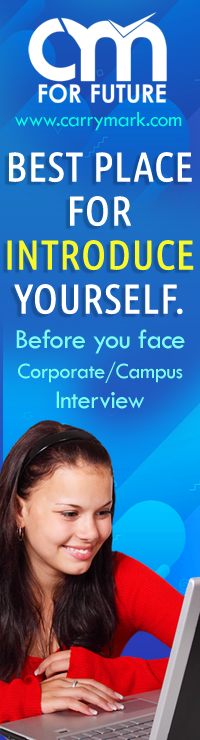