146. Python Program to find the Fibonacci series of a given number using Recursion.
How does this program work?
- This program is used to find the Fibonacci series of a given number using recursion in Python.
- Fibonacci series means the previous two elements are added to get the next element starting with 0 and 1.
- First we initializing first and second number as 0 and 1, and print them.
- The third number will be the sum of the first two numbers by using loop.
- By using Recursion method we can find the fibonacci series of a given number.
Here is the code
def fib(n):
if n <= 1:
return n
else:
return(fib(n-1) + fib(n-2))
nterms = int(input("Enter the number: "))
if nterms <= 0:
print("Plese enter a positive integer")
else:
print("Fibonacci sequence:")
for i in range(nterms):
print(fib(i), end=" ")
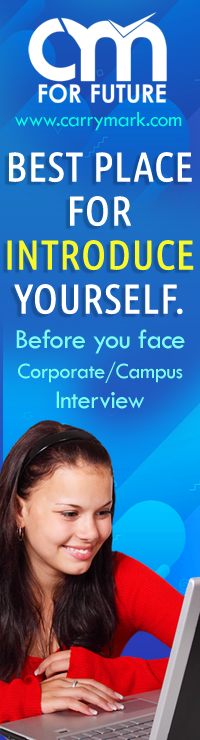