147. Python Program to find GCD of n numbers using Recursion.
How does this program work?
- This program is used to find GCD of n numbers using recursion in Python.
- GCD or HCF (Highest Common Factor) of numbers is the largest number that divides by them.
Here is the code
def gcd(a,b):
if(b==0):
return a
else:
return gcd(b,a%b)
a=int(input("Enter first number: "))
b=int(input("Enter second number: "))
GCD=gcd(a,b)
print("GCD of ",a, "and" ,b, "is: ")
print(GCD)
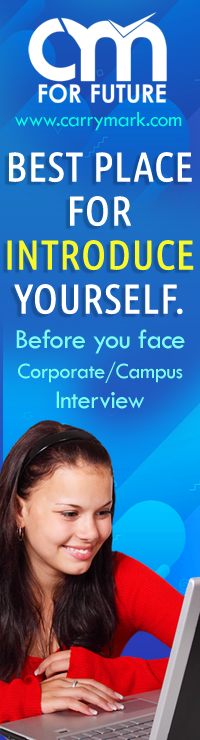